How to Format Unity's Time.time as hh:mm:ss
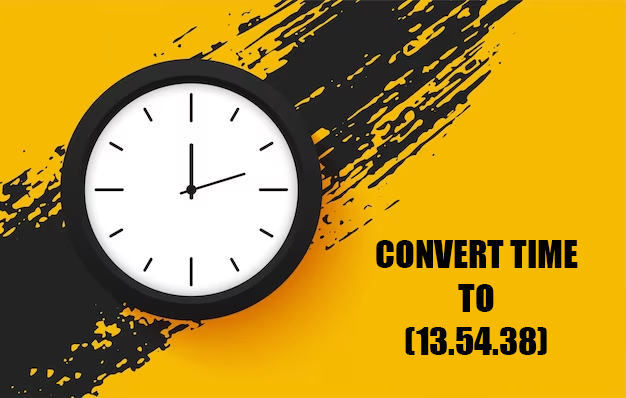
Unity provides developers with the Time.time
property, which returns the time in seconds since the game started. However, in many cases, you might want to display this time in a more user-friendly format, such as hours, minutes, and seconds (hh:mm:ss
). In this post, we’ll explore how to create a static function in Unity that takes the Time.time
value and formats it into a human-readable string.
Why Use Static Methods and Classes?
Before diving into the code, let’s talk about why we are using a static class and method. Static methods allow us to call a function without creating an instance of a class. This can be particularly useful when the method doesn’t need to store or manipulate instance-specific data. In this case, our formatting function simply processes the Time.time
value and returns a formatted string, making it an excellent candidate for a static approach.
Step 1: Creating the Static TimeExtensions
Class
We'll start by creating a new static class called TimeExtensions
. This class will contain a static method FormatAsTime()
that takes the Time.time
value and converts it into a hh:mm:ss
formatted string.
Here’s the code for the static class:
using UnityEngine;
public static class TimeExtensions
{
// Extension method for float to convert time to a specified format (hh:mm or hh:mm:ss)
public static string FormatAsTime(this float time, bool includeSeconds = false)
{
// Calculate hours, minutes, and seconds
int hours = Mathf.FloorToInt(time / 3600);
int minutes = Mathf.FloorToInt((time % 3600) / 60);
int seconds = Mathf.FloorToInt(time % 60);
// If includeSeconds is true, return hh:mm:ss, otherwise return hh:mm
if (includeSeconds)
{
return string.Format("{0:D2}:{1:D2}:{2:D2}", hours, minutes, seconds);
}
else
{
return string.Format("{0:D2}:{1:D2}", hours, minutes);
}
}
}
Breaking Down the Code:
-
Static Class:
public static class TimeExtensions
defines the class as static, meaning all its methods must also be static. You can't create instances of static classes. -
Static Method: The
FormatAsTime()
method is static, so it can be accessed directly through the class without needing an object. -
Time Calculation:
- The total time in seconds is retrieved via
Time.time
. - We calculate hours by dividing the time by
3600
(the number of seconds in an hour). - Minutes are derived from the remainder of the time divided by
3600
, and then dividing by60
. - Finally, seconds are calculated by taking the remainder of the total seconds divided by
60
.
- The total time in seconds is retrieved via
-
String Formatting: We use
string.Format()
to format the output ashh:mm:ss
, ensuring two digits for each value by using theD2
format specifier.
Step 2: Using the Static Method in Another Script
Now that we have our static TimeExtensions
class, let’s look at how we can use it in another script to display the formatted time.
In this example, we’ll use Unity's Debug.Log()
inside the Update()
method to print the formatted time every frame:
using UnityEngine;
public class ExampleUsage : MonoBehaviour
{
void Update()
{
// Example float time value
float time = Time.time;
// Log time in hh:mm:ss format
Debug.Log(time.FormatAsTime(includeSeconds: true));
// Log time in hh:mm format
Debug.Log(time.FormatAsTime(includeSeconds: false));
}
}
Why This Approach is Effective:
-
No Object Creation Needed: Since both the class and method are static, you don’t need to instantiate an object to access the method, simplifying the code.
-
Flexible Time Management: You can call
FormatAsTime()
from anywhere in your project where you need to display time, making the method highly reusable. -
Readable Output: Using the
hh:mm:ss
format is more user-friendly than displaying raw seconds, especially for games or apps that need to display elapsed time.
Conclusion
By using a static class and method in Unity, we’ve created a simple, efficient way to format the Time.time
property into hh:mm:ss
. This approach keeps our code clean, reusable, and easy to manage. Feel free to experiment with different time formats based on your project’s needs!