Colorizing Object Names in Unity's Hierarchy Window
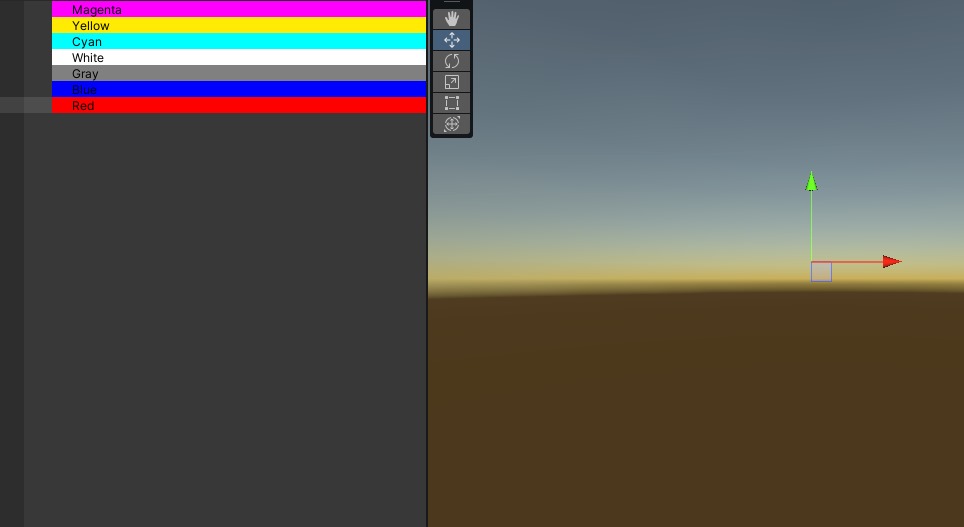
Unity's hierarchy window is essential for organizing your game objects, but sometimes it can be challenging to quickly identify specific objects. A great way to enhance visual organization is by colorizing the names of objects in the hierarchy. In this post, we’ll walk through creating a simple editor script that allows you to change the color of object names, making it easier to manage your scene.
Why Colorize Object Names?
Colorizing object names in the hierarchy can help you:
- Quickly identify different types of objects (e.g., enemies, props, lights).
- Organize your scene visually, especially in complex projects.
- Improve your workflow by reducing the time spent searching for objects.
Here is the code:
using UnityEngine;
using UnityEditor;
using System.Text.RegularExpressions;
#if UNITY_EDITOR
/// <summary>
/// Sets a background color for game objects in the Hierarchy tab based on the color specified in the GameObject's name.
/// </summary>
[InitializeOnLoad]
#endif
public class HierarchyObjectColor
{
private static readonly Vector2 Offset = new Vector2(20, 1);
static HierarchyObjectColor()
{
// Subscribe to the event to handle drawing in the Hierarchy window
EditorApplication.hierarchyWindowItemOnGUI += HandleHierarchyWindowItemOnGUI;
}
private static void HandleHierarchyWindowItemOnGUI(int instanceID, Rect selectionRect)
{
var obj = EditorUtility.InstanceIDToObject(instanceID) as GameObject;
if (obj != null)
{
Color backgroundColor = Color.clear;
Color textColor = Color.black;
// Determine the background color and text color based on the GameObject's name
switch (GetColorFromName(obj))
{
case "red":
backgroundColor = Color.red;
break;
case "gray":
backgroundColor = Color.gray;
break;
case "blue":
backgroundColor = Color.blue;
break;
case "cyan":
backgroundColor = Color.cyan;
break;
case "yellow":
backgroundColor = Color.yellow;
break;
case "green":
backgroundColor = Color.green;
break;
case "magenta":
backgroundColor = Color.magenta;
break;
case "white":
backgroundColor = Color.white;
break;
}
// Draw the background color if it's not clear
if (backgroundColor != Color.clear)
{
Rect bgRect = new Rect(selectionRect.x, selectionRect.y, selectionRect.width + 50, selectionRect.height);
EditorGUI.DrawRect(bgRect, backgroundColor);
// Draw the object name with the determined text color
Rect offsetRect = new Rect(selectionRect.position + Offset, selectionRect.size);
EditorGUI.LabelField(offsetRect, GetObjectName(obj), new GUIStyle
{
normal = new GUIStyleState { textColor = textColor }
});
}
}
}
private static string GetColorFromName(GameObject gameObject)
{
// Regex pattern to extract color name from the GameObject's name
var regex = new Regex("<color=(.*?)>", RegexOptions.IgnoreCase);
var match = regex.Match(gameObject.name);
// Return the color name if found, otherwise "clear"
return match.Success && match.Groups.Count >= 2 ? match.Groups[1].Value : "clear";
}
private static string GetObjectName(GameObject gameObject)
{
// Regex pattern to extract the text between color tags
var regex = new Regex(">(.*?)<", RegexOptions.IgnoreCase);
var match = regex.Match(gameObject.name);
// Return the extracted text if found, otherwise "clear"
return match.Success && match.Groups.Count >= 2 ? match.Groups[1].Value : "clear";
}
}
Step 2: Usage Instructions
Right-click in the Assets
panel, select Create > Folder, and name it Editor
.
Once the script is in place, you can colorize object names in the hierarchy by simply including the <color>
tag in the object's name. Here’s how to use it:
-
To set the name of an object to red, name it:
<color=red>My Enemy</color>
-
To set the name to green, use:
<color=green>Player Character</color>
-
You can use any color recognized by Unity’s color system, such as:
<color=blue>Item</color>
Conclusion
By utilizing the HierarchyObjectColor
script, you can easily colorize the names of objects in Unity's hierarchy window using simple color tags. This technique enhances organization and improves your overall development workflow. Feel free to experiment with different color schemes to see what works best for your project!